Use Google OpenID Authentication In Your ASP.NET With DotNetOpenAuth
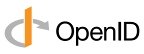
On this article, I will show you a ASP.NET sample code I made that use OpenID Authentication to verify Google Account. To accomplish the authentication, I used the C# library called DotNetOpenAuth. Here is the step-by-step procedure to implement it on your ASP.NET application.
1. Download the DotNetOpenAuth Libraries. Choose the most appropriate version for development platform.
2. Extract the downloaded compressed file on your hard drive.
3. On your project, Add Reference to "DotNetOpenAuth.dll"
4. On your login page's HTML Code, paste the following.
- <form id="form1" runat="server">
- <div id="loginform">
- <div id="NotLoggedIn" runat="server">
- Log in with <img src="http://www.google.com/favicon.ico" />
- <asp:Button ID="btnLoginToGoogle" Runat="server" Text="Google" OnCommand="OpenLogin_Click"
- CommandArgument="https://www.google.com/accounts/o8/id" />
- <p /><asp:Label runat="server" ID="lblAlertMsg" />
- </div>
- </div>
- </form>
Take note of the URL: https://www.google.com/accounts/o8/id - this is the unique OpenID URL of Google Account.
5. Include the following namespaces on your "Using" directive.
- using DotNetOpenAuth.OpenId;
- using DotNetOpenAuth.OpenId.RelyingParty;
6. On the Page_Load & OpenLogin_Click, use the following codes.
- protected void Page_Load(object sender, EventArgs e)
- {
- OpenIdRelyingParty rp = new OpenIdRelyingParty();
- var r = rp.GetResponse();
- if (r != null)
- {
- switch (r.Status)
- {
- case AuthenticationStatus.Authenticated:
- NotLoggedIn.Visible = false;
- Session["GoogleIdentifier"] = r.ClaimedIdentifier.ToString();
- Response.Redirect("Main.aspx"); //redirect to main page of your website
- break;
- case AuthenticationStatus.Canceled:
- lblAlertMsg.Text = "Cancelled.";
- break;
- case AuthenticationStatus.Failed:
- lblAlertMsg.Text = "Login Failed.";
- break;
- }
- }
- protected void OpenLogin_Click(object src, CommandEventArgs e)
- {
- string discoveryUri = e.CommandArgument.ToString();
- OpenIdRelyingParty openid = new OpenIdRelyingParty();
- var b = new UriBuilder(Request.Url) { Query = "" };
- var req = openid.CreateRequest(discoveryUri, b.Uri, b.Uri);
- req.RedirectToProvider();
- }
7. Run the project. It should look like the following screens.
a. Login page
b. Main Page (showing the returned identifier by Google)
No comments:
Post a Comment